Splines in Unreal Engine are essential tool for almost anything! in this post we are covering Design , Code and Everything in between:
Table Of Content
Section | Description |
---|---|
1. Introduction | Overview of splines in Unreal Engine and why they are useful. |
2. Core Concepts of Splines in Unreal Engine | Explanation of control points, tangents, and mesh deformation. |
2.1 Understanding Spline Components | USplineComponent (stores paths) vs. USplineMeshComponent (deforms meshes). |
3. Top Use Cases for Splines | Common applications like roads, rivers, camera paths, and AI navigation. |
3.1 Environment Design | Using splines for level design (roads, fences, rivers). |
3.2 Camera & Cinematics | Creating smooth camera movements and cutscene rails. |
3.3 Dynamic Gameplay | Moving platforms, procedural paths, and AI patrol routes. |
4. Common Issues & Optimization | Fixing texture stretching, mesh twisting, and performance concerns. |
4.1 Texture & Mesh Issues | How to fix texture stretching and misaligned meshes. |
4.2Rotation/Twisting of Spline Meshes | When to use the Merge Actors Tool to convert splines into static meshes. |
4.3 Splines Causing FPS Drops | Simply Dont Use spline in Runtime! |
5. Developer’s Guide: Runtime Spline Updates | Best practices for controlling splines in gameplay. |
5.1Unreal’s Cooking System & TMap Pitfall | Using GetLocationAtDistanceAlongSpline() efficiently. |
5.2Using TObjectPtr for Meshes & Materials in Splines | How to properly modify spline meshes in-game. |
5.3Ensuring Spline Members Show in Both Editor & Packaged Game
Runtime Movement Spline Basef |
Why TMap for meshes/materials won’t cook and how to fix it with TObjectPtr . |
6. Conclusion | Summary of best practices for designers and developers. |
1.Introduction
Splines in Unreal Engine are one of the most versatile tools for both designers and programmers. If you’ve ever used the Pen Tool in Photoshop or Illustrator, you’ll feel right at home—splines let you draw smooth, customizable curves in 3D space, perfect for guiding objects, creating roads, or even designing entire gameplay systems.
For designers, splines offer an intuitive way to shape environments—whether it’s carving a winding river through a landscape, laying down a fence along a path, or animating a cinematic camera movement. The best part? You can tweak and adjust them entirely in the editor without writing a single line of code.
For programmers, splines unlock a range of powerful procedural techniques. With a few lines of code, you can dynamically generate paths, control AI movement, or even simulate physics-based ropes and chains. This article will guide you through everything from basic spline editing to advanced runtime usage, with dedicated sections for designers and optional deep dives for coders.
2.UseCases: Why Are Splines So Powerful?
Splines are an essential tool in Unreal Engine because they provide a flexible way to define movement paths, shape assets, and create dynamic environments. Here are just a few examples of how they are used in game development:
- Environment Art & Level Design – Quickly create roads, rivers, fences, bridges, and natural terrain features by stretching meshes along a spline.
- Cinematic Camera Paths – Define smooth, controlled camera movements for cutscenes or player-guided sequences.
- AI Navigation & Patrol Routes – Create dynamic paths for enemy patrols or NPC movement.
- Dynamic Gameplay Elements – Use splines to control moving platforms, swinging ropes, or even procedural animations.
- Procedural Generation – Generate entire game elements like power lines, railroads, or randomized dungeon layouts at runtime.
- Particle & FX Control – Direct particles along a predefined path for visual effects like smoke trails, magic spells, or firework sequences.
- Physics Simulations – Use splines to generate ropes, cables, or chains that interact with gravity and external forces.
Each of these use cases can be approached in a designer-friendly way inside Unreal’s editor tools, but programmers can also extend them further by dynamically modifying splines at runtime. In the next sections, we’ll explore both approaches, ensuring that whether you’re an artist, level designer, or developer, you’ll be able to leverage splines effectively in your projects.
2.1 Spline Concepts in Unreal Engine
The Photoshop Analogy: Understanding Splines Visually
If you’ve ever worked with the Pen Tool in Photoshop or Illustrator, you already understand the core of Unreal’s splines. A spline is a curved path defined by control points, and just like in vector graphics, these points influence the shape of the curve:
Control Points – The main points that define the path. Adjusting their position shapes the spline.
Tangents (Bezier Handles) – Invisible directional lines that control the smoothness of the curve.
Spline Mesh Components – Allow you to stretch static meshes along a spline, great for things like roads and pipes.
This means you can use splines to define precise, customizable paths without manually placing every detail—making them one of the most efficient tools in Unreal Engine for both static and dynamic elements.
Key Unreal Engine Spline Components
Splines in Unreal are managed through two main components:
-
1. USplineComponent (Stores the path)
- Defines a curve with adjustable control points.
- Used for things like AI paths, player movement guides, and visual effects.
-
2. USplineMeshComponent (Meshes along the spline)
- Allows static meshes (e.g., a road or pipe) to follow the curve.
- Great for procedural roads, fences, power lines, or bridges.
With these two components, you can create powerful tools for environment design and gameplay mechanics.
3.Top Spline Use Cases (With Workflows)
Splines are widely used in game development, offering both editor-side convenience for designers and runtime flexibility for programmers. Below are some of the most common use cases:
1. Environment Art: Creating Roads, Rivers, and Fences
- Designer Workflow:
- Place a spline in the level and adjust the control points to follow the terrain.
- Attach a Spline Mesh Component and assign a road, river, or fence mesh.
- Fine-tune the width and UV tiling to prevent texture stretching.
- Done! The environment is built with minimal effort and maximum flexibility.
2. Cinematic Camera Paths
- Designer Workflow:
- Create a spline and attach a Cine Camera Actor to it.
- Use Sequencer to animate the camera’s movement along the spline.
- Adjust speed and easing for smooth dolly shots or tracking movements.
3. AI Navigation & Patrol Routes
- Programmer Workflow:
- Define patrol paths using splines and move AI characters along them dynamically.
- Example: A guard follows a predefined route, stopping at key points before continuing.
FVector PatrolLocation = SplineComponent->GetLocationAtDistanceAlongSpline(Distance, ESplineCoordinateSpace::World);
AICharacter->SetActorLocation(PatrolLocation);
- Designers draw a looping spline in the editor.
- Programmers set up a Timer-based movement system for better performance than Tick.
- The platform smoothly moves along the spline at runtime.
5. Procedural Generation: Roads, Railways, and Dungeon Layouts
- Programmer Workflow:
- Use splines to generate environments dynamically.
- Example: A road automatically spawns meshes and follows terrain elevation.
6. Particle & FX Control: Fireworks, Magic Trails, and Smoke Paths
Designer Workflow:
-
- Use a spline to guide Niagara particles for effects like a dragon breathing fire along a curved path.
- Adjust spline curvature for more natural movement
4.Common Issues & Optimization Tips for Splines
Splines in Unreal Engine are incredibly useful, but they come with certain performance considerations. Here’s how to avoid common pitfalls and optimize your splines efficiently.
Common Issues & How to Fix Them
4.1 Texture Stretching on Spline Meshes
Problem: When stretching a mesh along a spline (e.g., a road or pipe), textures can appear stretched or squashed.
Solution:
Adjust the UV tiling in the Spline Mesh Component (SetStartScale() and SetEndScale() can help).
Ensure the Static Mesh’s UV mapping is suitable for tiling.
Alternatively, use a Material with World Aligned Textures to prevent stretching.
4.2 Rotation/Twisting of Spline Meshes
Problem: The spline mesh appears twisted, or its orientation does not align with the path.
Solution:
Adjust the Forward Axis setting in the Spline Mesh Component (try X-axis or Z-axis depending on the mesh).
Manually set rotation using:
SplineMesh->SetStartAndEnd(StartPos, StartTangent, EndPos, EndTangent);
If the mesh still twists, consider breaking it into smaller segments for smoother transitions.
4.3 Performance Issues: Splines Causing FPS Drops
Problem: A large number of splines updated every frame can impact performance, especially when used for AI movement, physics objects, or dynamic environments.
Solution:
If your spline is only deforming meshes and not being used in runtime, don’t worry about performance!
Simply use Unreal’s “Merge Actors” tool to convert the spline mesh into a static mesh.
This is a tricky but powerful optimization—it prevents unnecessary calculations and makes the spline behave like a normal mesh.
If your spline needs to move or update during runtime:
Use Timers Instead of Tick (for moving platforms, AI, etc.):
GetWorldTimerManager().SetTimer(UpdateTimer, this, &AMovingPlatform::UpdateSplineMovement, 0.05f, true);
Only Update Splines When Necessary (e.g., when the player is near):
if (FVector::Dist(Player->GetActorLocation(), Spline->GetComponentLocation()) < 500.0f)
{
UpdateSplineLogic();
}
Reduce Spline Point Density: Fewer points mean fewer calculations.
And if your spline logic is simple, don’t bother with coding!
The Spline Mesh Component can move during runtime and is useful in many cases.
Great for animated ropes, conveyor belts, or simple dynamic elements.
4. AI Moving Too Fast or Stopping Abruptly on Splines
Problem: AI characters moving along splines either speed up unexpectedly or stop suddenly at spline points.
Solution:
Use a distance-based movement system instead of a time-based system:
float Distance = Speed * DeltaTime;
FVector NewLocation = SplineComponent->GetLocationAtDistanceAlongSpline(Distance, ESplineCoordinateSpace::World);
AICharacter->SetActorLocation(NewLocation);
If needed, use GetTangentAtDistanceAlongSpline() to smoothly adjust rotation.
5. Spline Paths Not Aligning Properly When Edited
Problem: When editing spline points, objects following the spline sometimes behave unpredictably.
Solution:
Always call UpdateSpline() after modifying a spline at runtime:
SplineComponent->UpdateSpline();
Ensure rotation follows the spline’s forward vector using:
FRotator Rotation = SplineComponent->GetRotationAtDistanceAlongSpline(Distance, ESplineCoordinateSpace::World);
Actor->SetActorRotation(Rotation);
Optimization Tips for Splines in Large-Scale Projects
1. Use Object Pooling for Spline Meshes
Instead of spawning new spline mesh components every time, reuse existing ones.
Helps when dynamically generating roads, fences, or train tracks.
2. Reduce Tick Usage for Moving Objects
Avoid updating spline movement every frame—use Timers or distance-based updates.
3. Disable Unnecessary Collision on Spline Meshes
If a spline mesh doesn’t require physics interactions, disable collision to improve performance:
SplineMesh->SetCollisionEnabled(ECollisionEnabled::NoCollision);
4. Use LODs for Complex Spline-Based Structures
If using splines for roads, pipes, or procedural geometry, use Level of Detail (LOD) meshes to reduce render cost.
Important Note for Coders: Don’t Use TMap for Spline Data!
Many developers store spline data in TMap structures to associate values with points (e.g., storing speed values per control point). However, TMaps are not cooked in the shipped version of Unreal Engine, meaning your game might work fine in the editor but break in the final build!
Instead, use arrays (TArray) or structured data (FStructs) to store spline-related values.
Example of a safe way to store per-point data:
TArray<float> SpeedPerPoint; // Stores speed values per spline point
5.Developers’ Section: Advanced Spline Techniques & Important Unreal Engine Gotchas
This section is for developers who want to take splines beyond simple editor use—whether it’s procedural generation, runtime modifications, or AI navigation. Here, we’ll cover best practices and common pitfalls to avoid in Unreal Engine.
1. Unreal’s Cooking System & TMap Pitfall
If you’re using TMap to store spline-related data, be careful!
TMap is not cooked into the shipped build by default. This means your spline-related mappings (e.g., associating control points with materials, speeds, or object references) might work fine in the editor but will be empty in the packaged game.
This is a common issue that breaks runtime spline logic in shipped builds.
Solution:
Instead of TMap, use TArray or TMap wrapped in UPROPERTY().
Alternatively, use FStructs with arrays inside them:
USTRUCT(BlueprintType)
struct FSplineData
{
GENERATED_BODY()
UPROPERTY(EditAnywhere, BlueprintReadWrite)
float Speed;
UPROPERTY(EditAnywhere, BlueprintReadWrite)
UMaterialInterface* Material;
};
UPROPERTY(EditAnywhere, BlueprintReadWrite)
TArray<FSplineData> SplineDataArray; // Stores speed & materials per point
This ensures the data is properly saved and available in shipped builds.
2. Using TObjectPtr for Meshes & Materials in Splines
If your spline needs to store materials, meshes, or other assets, do not use raw pointers (UStaticMesh* or UMaterial*). Instead, use TObjectPtr<>, which is optimized for Unreal’s garbage collection and asset management.
Example (Correct Way):
UPROPERTY(EditAnywhere, BlueprintReadWrite)
TObjectPtr<UStaticMesh> RoadMesh;
This avoids crashes and ensures proper asset referencing in packaged builds.
3. Ensuring Spline Members Show in Both Editor & Packaged Game
If you declare variables like this:
TArray<FVector> SplinePoints;
They will be visible in the editor, but in a cooked build, they will be empty!
Solution:
Always mark spline-related variables with
UPROPERTY(EditAnywhere)
to ensure they are properly serialized and available in the packaged game.
Example:
UPROPERTY(EditAnywhere, BlueprintReadWrite)
TArray<FVector> SplinePoints;
This ensures that the spline’s points persist in a cooked build.
Controlling Spline Mesh Component Movement at Runtime in Unreal Engine
If you want to move a spline mesh dynamically during gameplay, Unreal provides powerful built-in functions. Instead of manually adjusting each vertex, you should update the Spline Mesh Component directly using Unreal’s built-in methods.
1. Understanding How Spline Mesh Movement Works
A USplineMeshComponent is a segment between two spline points. When moving the spline dynamically in runtime, you need to update:
Start & End Positions
Tangents for Smooth Curves
Spline Mesh Components (if adding new ones dynamically)
💡 Important Note:
UpdateSpline() only updates the USplineComponent (the path), but it does NOT automatically update USplineMeshComponent instances.
You must manually refresh each spline mesh component after modifying the spline points.
2. Moving a Spline Mesh at Runtime (Correct Way)
- A. Move an Existing Spline and Update Meshes
If you want to modify a spline’s shape dynamically (e.g., a moving bridge, conveyor belt, or shifting road):
✅ Best Approach: Adjust Spline Mesh Start & End Points
void AMySplineActor::MoveSplineMesh(float DeltaTime)
{
if (!SplineComponent || SplineMeshes.Num() == 0) return;
// Example: Move the first spline point forward
FVector NewLocation = SplineComponent->GetLocationAtSplinePoint(0, ESplineCoordinateSpace::World);
NewLocation += FVector(100 * DeltaTime, 0, 0); // Move right over time
SplineComponent->SetLocationAtSplinePoint(0, NewLocation, ESplineCoordinateSpace::World);
SplineComponent->UpdateSpline();
// Update all spline mesh components
for (int32 i = 0; i < SplineMeshes.Num(); i++)
{
FVector StartLocation = SplineComponent->GetLocationAtSplinePoint(i, ESplineCoordinateSpace::World);
FVector StartTangent = SplineComponent->GetTangentAtSplinePoint(i, ESplineCoordinateSpace::World);
FVector EndLocation = SplineComponent->GetLocationAtSplinePoint(i + 1, ESplineCoordinateSpace::World);
FVector EndTangent = SplineComponent->GetTangentAtSplinePoint(i + 1, ESplineCoordinateSpace::World);
SplineMeshes[i]->SetStartAndEnd(StartLocation, StartTangent, EndLocation, EndTangent);
}
}
✅ Why This Works Well
Efficient: Only updates spline meshes when necessary.
Smooth movement: Ensures proper tangents so meshes don’t “snap.”
Works without re-creating spline meshes, keeping performance high.
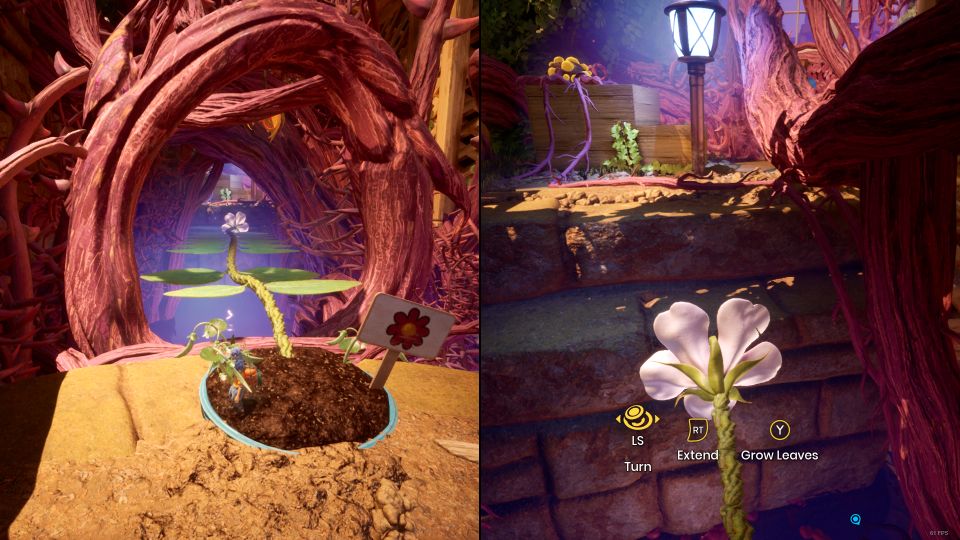
- B. Animate a Spline Mesh Moving Along a Path
If you want an object (like a train, vehicle, or enemy) to move along the spline instead of deforming it, use:
✅ Move Actor Along a Spline Over Time
void AMyMovingActor::MoveAlongSpline(float DeltaTime)
{
if (!SplineComponent) return;
DistanceAlongSpline += Speed * DeltaTime; // Increment movement
float SplineLength = SplineComponent->GetSplineLength();
DistanceAlongSpline = FMath::Fmod(DistanceAlongSpline, SplineLength); // Loop back
FVector NewLocation = SplineComponent->GetLocationAtDistanceAlongSpline(DistanceAlongSpline, ESplineCoordinateSpace::World);
FRotator NewRotation = SplineComponent->GetRotationAtDistanceAlongSpline(DistanceAlongSpline, ESplineCoordinateSpace::World);
SetActorLocationAndRotation(NewLocation, NewRotation);
}
✅ Why This Works Well
No need to update the spline itself—only the moving actor.
Uses GetLocationAtDistanceAlongSpline() instead of manually interpolating movement.
Works with AI, vehicles, cameras, and platforms.
- C. Create Spline Meshes Dynamically (For Procedural Spline Systems)
If you’re generating splines at runtime (e.g., drawing a custom path for roads or rivers), you must manually spawn USplineMeshComponent instances:
void AMySplineActor::GenerateSplineMeshes()
{
if (!SplineComponent) return;
// Clear existing meshes
for (USplineMeshComponent* SplineMesh : SplineMeshes)
{
SplineMesh->DestroyComponent();
}
SplineMeshes.Empty();
// Loop through spline points and create meshes
int32 NumPoints = SplineComponent->GetNumberOfSplinePoints();
for (int32 i = 0; i < NumPoints - 1; i++)
{
USplineMeshComponent* NewSplineMesh = NewObject<USplineMeshComponent>(this);
NewSplineMesh->RegisterComponent();
NewSplineMesh->AttachToComponent(SplineComponent, FAttachmentTransformRules::KeepRelativeTransform);
FVector StartLocation = SplineComponent->GetLocationAtSplinePoint(i, ESplineCoordinateSpace::World);
FVector StartTangent = SplineComponent->GetTangentAtSplinePoint(i, ESplineCoordinateSpace::World);
FVector EndLocation = SplineComponent->GetLocationAtSplinePoint(i + 1, ESplineCoordinateSpace::World);
FVector EndTangent = SplineComponent->GetTangentAtSplinePoint(i + 1, ESplineCoordinateSpace::World);
NewSplineMesh->SetStaticMesh(RoadMesh);
NewSplineMesh->SetStartAndEnd(StartLocation, StartTangent, EndLocation, EndTangent);
SplineMeshes.Add(NewSplineMesh);
}
}
✅ Why This Works Well
- Dynamically creates spline meshes during gameplay.
- Allows runtime procedural generation of roads, fences, tracks, etc.
- Avoids spawning excessive actors—all meshes exist within one actor.
3. Performance Best Practices
If you’re modifying splines frequently at runtime, keep these performance optimizations in mind:
✅ Use Spline Mesh Component Instead of Custom Mesh Deformation
Unreal’s built-in USplineMeshComponent already supports movement and deformation without re-creating mesh instances.
✅ Don’t Overuse Tick(), Use Timers Instead
Instead of updating every frame, use a Timer to update movement at fixed intervals (e.g., 10-20 times per second):
GetWorldTimerManager().SetTimer(MoveTimer, this, &AMySplineActor::MoveSplineMesh, 0.05f, true);
✅ Batch Spline Updates
Instead of updating one point per frame, modify multiple points, then call UpdateSpline() once.
✅ Avoid Over-Adding Spline Mesh Components
If adding/removing spline meshes dynamically, reuse existing components instead of constantly destroying and spawning new ones.
✅ For Fixed Splines (Non-Moving), Convert to Static Mesh
If your spline won’t move at runtime, use Unreal’s “Merge Actors” tool to bake it into a static mesh for better performance.
Final Thoughts: When to Use What?
- By following these best practices, your splines will be smooth, performant, and fully functional in both editor and shipped builds!
6.Conclusion: Mastering Splines in Unreal Engine
Splines in Unreal Engine are a powerful and flexible tool for both designers and developers. Whether you’re creating roads, rivers, camera paths, or dynamic gameplay elements, understanding when to use splines and how to optimize them is crucial. Here’s a summary of key takeaways from this guide:
1. Core Concepts of Splines
✅ Splines are like Photoshop’s Pen Tool – use control points and tangents to shape curves.
✅ Spline Components store path data, while Spline Mesh Components deform meshes along them.
✅ Best Use Cases include roads, AI paths, procedural fences, moving platforms, and camera rails.
2. Common Issues & Optimization Tips
✅ If you’re not modifying splines in-game, use Merge Actors Tool to convert them into static meshes for performance.
✅ Texture stretching? Adjust UV tiling or tweak the spline mesh’s texture settings.
✅ Spline meshes twisting? Set proper forward and up axes in Spline Mesh Component.
✅ Updating splines dynamically? Avoid OnConstruction() (only works in the editor) – use UpdateSpline() at runtime.
✅ Moving objects along a spline? Use GetLocationAtDistanceAlongSpline() instead of manually interpolating movement.
✅ For large spline-based systems, use timers instead of Tick() to improve performance.
3. Developer-Specific Notes
✅ For runtime spline systems, avoid using TMap for meshes or materials – they won’t be cooked in a shipped build. Instead, use TObjectPtr<UStaticMesh> and mark important variables with UPROPERTY(EditAnywhere).
✅ Don’t overcomplicate things – USplineMeshComponent already supports movement at runtime, so in many cases, there’s no need for custom deformation code.
Designers: Splines are your best tool for fast level design and smooth organic shapes.
Developers: Use optimized methods to modify splines in runtime, and avoid common pitfalls like using OnConstruction() or TMaps incorrectly.
Performance Matters: If a spline doesn’t need runtime updates, bake it into a static mesh for better efficiency.
By following these best practices, your spline-based systems will be efficient, easy to modify, and work smoothly in shipped builds!